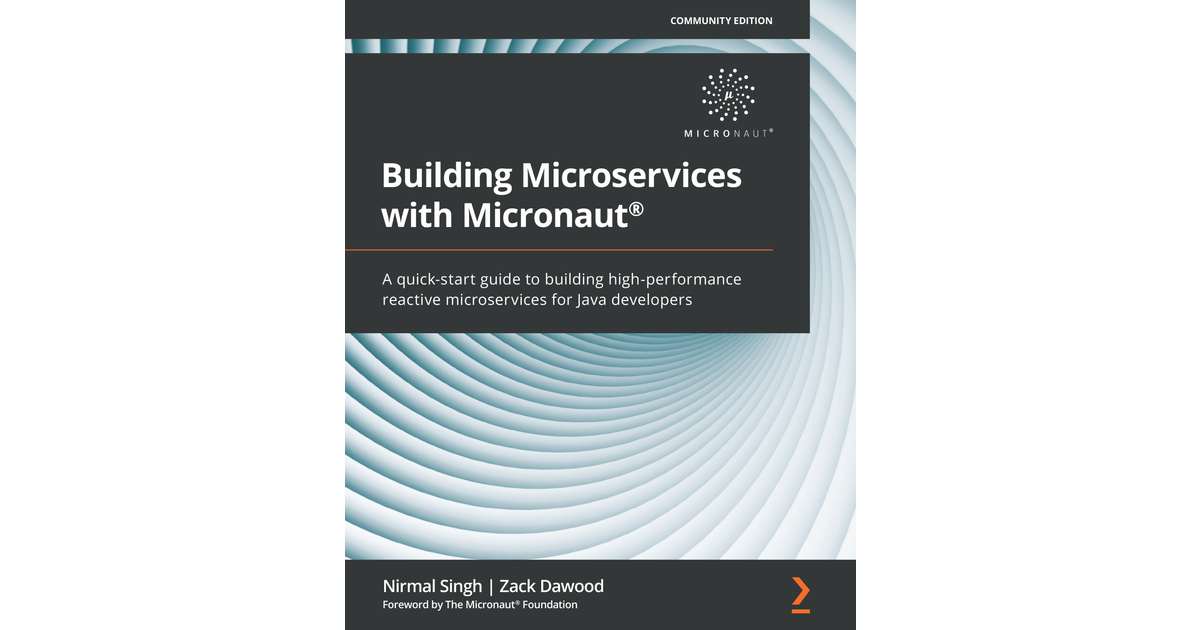
Building micronaut microservices using microstartercli
Building Micronaut microservices using the Microstarter CLI is a streamlined process that involves leveraging a command-line interface to quickly scaffold and set up Micronaut projects. Below are the steps and essential information to get you started:
Prerequisites
- Java Development Kit (JDK): Ensure you have JDK 8 or later installed.
- Microstarter CLI: Install Microstarter CLI.
Installing Microstarter CLI
You can install Microstarter CLI using SDKMAN, a tool for managing parallel versions of multiple Software Development Kits:
$ sdk install microstarter
Creating a Micronaut Project
Once Microstarter CLI is installed, you can create a new Micronaut project:
$ microstarter create-app <project-name>
Replace <project-name>
with your desired project name. This command scaffolds a new Micronaut application with the basic structure.
Project Structure
A typical Micronaut project created by Microstarter CLI includes:
src/main/java
: The main application source code.src/test/java
: The test code.src/main/resources
: Configuration files.build.gradle
orpom.xml
: The build file (Gradle or Maven).
Configuring Build Tool
Depending on your preference, you can choose Gradle or Maven as your build tool.
Using Gradle
Ensure build.gradle
contains necessary dependencies:
plugins {
id 'io.micronaut.application' version '1.0.0'
}
dependencies {
annotationProcessor "io.micronaut:micronaut-inject-java"
implementation "io.micronaut:micronaut-runtime"
implementation "javax.annotation:javax.annotation-api"
testImplementation "io.micronaut:micronaut-http-client"
}
micronaut {
runtime "netty"
testRuntime "junit5"
processing {
incremental true
annotations "your.package.*"
}
}
Using Maven
Ensure pom.xml
is properly configured:
<dependencies>
<dependency>
<groupId>io.micronaut</groupId>
<artifactId>micronaut-runtime</artifactId>
</dependency>
<dependency>
<groupId>io.micronaut</groupId>
<artifactId>micronaut-http-client</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>javax.annotation</groupId>
<artifactId>javax.annotation-api</artifactId>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>io.micronaut.maven</groupId>
<artifactId>micronaut-maven-plugin</artifactId>
<version>1.0.0</version>
</plugin>
</plugins>
</build>
Creating Controllers
Generate a controller using the CLI:
$ microstarter create-controller com.example.HelloController
This command creates a controller class in src/main/java/com/example/HelloController.java
.
Example Controller
Here’s an example of a simple controller:
package com.example;
import io.micronaut.http.annotation.Controller;
import io.micronaut.http.annotation.Get;
public class HelloController {
public String index() {
return "Hello, World!";
}
}
Running the Application
You can run the application using:
$ ./gradlew run # For Gradle
$ mvn mn:run # For Maven
Testing
Micronaut applications typically use JUnit 5 for testing. Example test case:
package com.example;
import io.micronaut.http.client.HttpClient;
import io.micronaut.http.client.annotation.Client;
import io.micronaut.test.extensions.junit5.annotation.MicronautTest;
import org.junit.jupiter.api.Test;
import javax.inject.Inject;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class HelloControllerTest {
HttpClient client;
void testHelloEndpoint() {
String response = client.toBlocking().retrieve("/hello");
assertEquals("Hello, World!", response);
}
}
Deploying
Micronaut applications can be deployed to various platforms including:
- Docker: Create a Dockerfile to containerize your application.
- Kubernetes: Deploy the Docker container to a Kubernetes cluster.
- Cloud Providers: Use providers like AWS, Google Cloud, or Azure.
Example Dockerfile
FROM openjdk:11-jre-slim
COPY build/libs/your-application.jar /app.jar
ENTRYPOINT ["java", "-jar", "/app.jar"]
Build the Docker image:
$ docker build -t your-application .
Run the Docker container:
$ docker run -p 8080:8080 your-application
Conclusion
Using Microstarter CLI with Micronaut significantly reduces the boilerplate and setup time for building microservices. This guide should help you get started quickly with your first Micronaut microservice. For more advanced configurations and features, refer to the official Micronaut documentation.