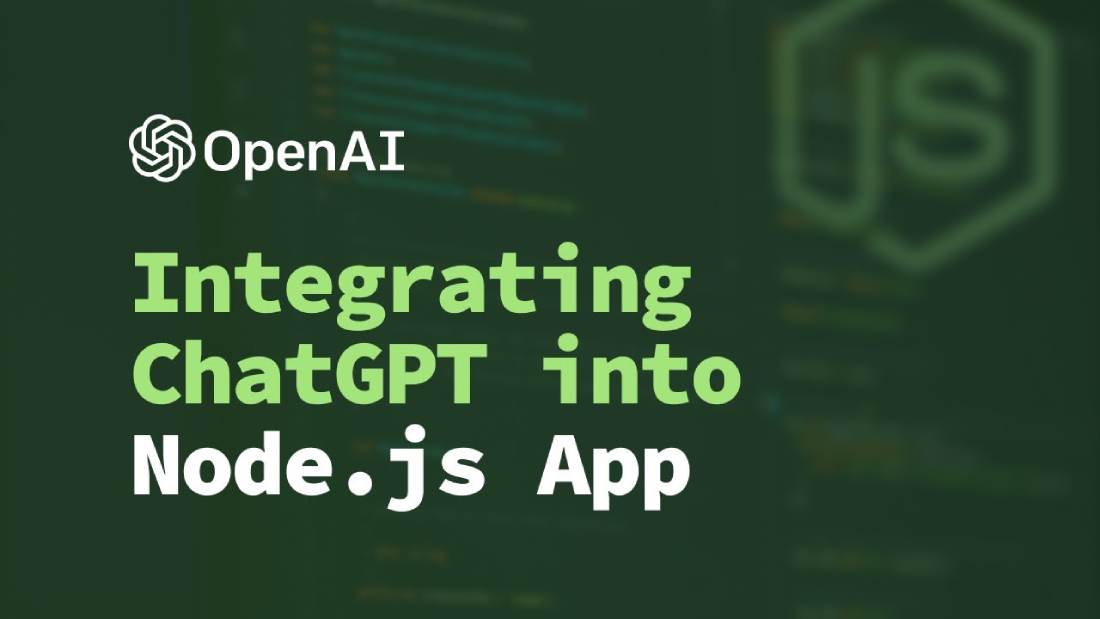
How to Use OpenAI’s ChatGPT API in Node.js
How to Use OpenAI’s ChatGPT API in Node.js: OpenAI’s ChatGPT API allows developers to integrate the powerful language generation capabilities of the GPT-3.5 model into their Node.js applications. You can build chatbots, virtual assistants, customer support systems, and more with the API. In this article, we’ll explore how to use the ChatGPT API in Node.js to create dynamic conversational experiences.
Prerequisites
Before we begin, make sure you have the following prerequisites set up:
- Node.js: Install Node.js on your development machine. You can download it from the official website (https://nodejs.org) and follow the installation instructions for your operating system.
- OpenAI Account: Sign up for an account on the OpenAI website (https://openai.com) if you don’t have one already. This is required to obtain an API key for accessing the ChatGPT API.
- OpenAI API Key: Retrieve your OpenAI API key from the OpenAI website. You can find your API key under your account dashboard’s “API Keys” section.
Setting up the Project
Once you have the prerequisites ready, follow these steps to set up your Node.js project:
- Create a new directory for your project and navigate to it in the terminal.
- Initialize a new Node.js project by running the following command:
csharp
npm init -y
This will create a
package.json
file that tracks the dependencies and configurations for your project. - Install the
axios
package, which we’ll use to make HTTP requests to the ChatGPT API. Run the following command:npm install axios
This will add the
axios
package as a dependency to your project. - Create a new JavaScript file, such as,
chatbot.js
and open it in your preferred code editor.
Making Requests to the ChatGPT API
In your chatbot.js
file, you can start writing the code to interact with the ChatGPT API. Here’s a basic example to get you started:
const axios = require('axios');
const apiKey = ‘YOUR_API_KEY’;
const apiUrl = ‘https://api.openai.com/v1/engines/davinci-codex/completions’;
async function chatWithGPT3(prompt) {
try {
const response = await axios.post(apiUrl, {
prompt: prompt,
max_tokens: 50,
}, {
headers: {
‘Content-Type’: ‘application/json’,
‘Authorization’: `Bearer ${apiKey}`,
},
});
const { choices } = response.data;
const reply = choices[0].text.trim();
return reply;
} catch (error) {
console.error(‘Error:’, error);
throw error;
}
}
// Example usage
async function main() {
const prompt = ‘What is the meaning of life?’;
const reply = await chatWithGPT3(prompt);
console.log(‘ChatGPT API Reply:’, reply);
}
main();
In the above code, we import the axios
package and define the API key and API URL. Replace 'YOUR_API_KEY'
with your actual OpenAI API key.
The chatWithGPT3
the function takes a prompt as input, makes a POST request to the ChatGPT API, and retrieves the generated reply from the response. We use axios.post
to send the request, including the prompt and the desired max_tokens
response.
In the main
function, you can call chatWithGPT3
with your desired prompt and log the reply to the console.
How to Use OpenAI’s ChatGPT API in Node.js
Customizing the ChatGPT Behavior
The example above uses the base davinci-codex
engine and sets max_tokens
to 50. You can modify these settings according to your needs. Additionally, you can explore various ChatGPT API parameters to customize the behavior and fine-tune the responses.
Conclusion
This article explored how to use OpenAI’s ChatGPT API in Node.js to create dynamic conversational experiences. By following the steps outlined, you can integrate the power of the ChatGPT model into your applications and build sophisticated chatbots and virtual assistants. Remember to keep your API key secure and refer to the official OpenAI documentation for more details on available options and best practices. Happy coding!